How To Learn React As A Beginner In 2024

Let’s be frank: building a website or web app with a truly interactive and responsive user interface can be challenging. You may or may not have experience with HTML, CSS, and JavaScript, and creating dynamic UI elements that remain fast and fluid is difficult.
This is where React comes in.
React simplifies the process of building a modern, interactive user interface (UI) compared to traditional methods by leveraging a component-based architecture.
User Interface
User Interface (UI) refers to the point where humans interact with computers on web pages, device, or apps. It’s a web design term focusing on user engagement.
Read More
This allows you to create reusable code and utilize a virtual Document Object Model (or DOM) that renders UI changes at lightning speed.
But where do you even begin? In this short guide, we’ve compiled the best resources for anyone who wants to learn React. We’ll look at interactive coding platforms, comprehensive video courses, and hands-on projects, all designed with the beginner in mind. Let’s get started!
What Is React Js?
React is a wildly popular JavaScript library currently used by over 40% of all JavaScript developers, second to only Node.js, which is used by 42.65% of devs.
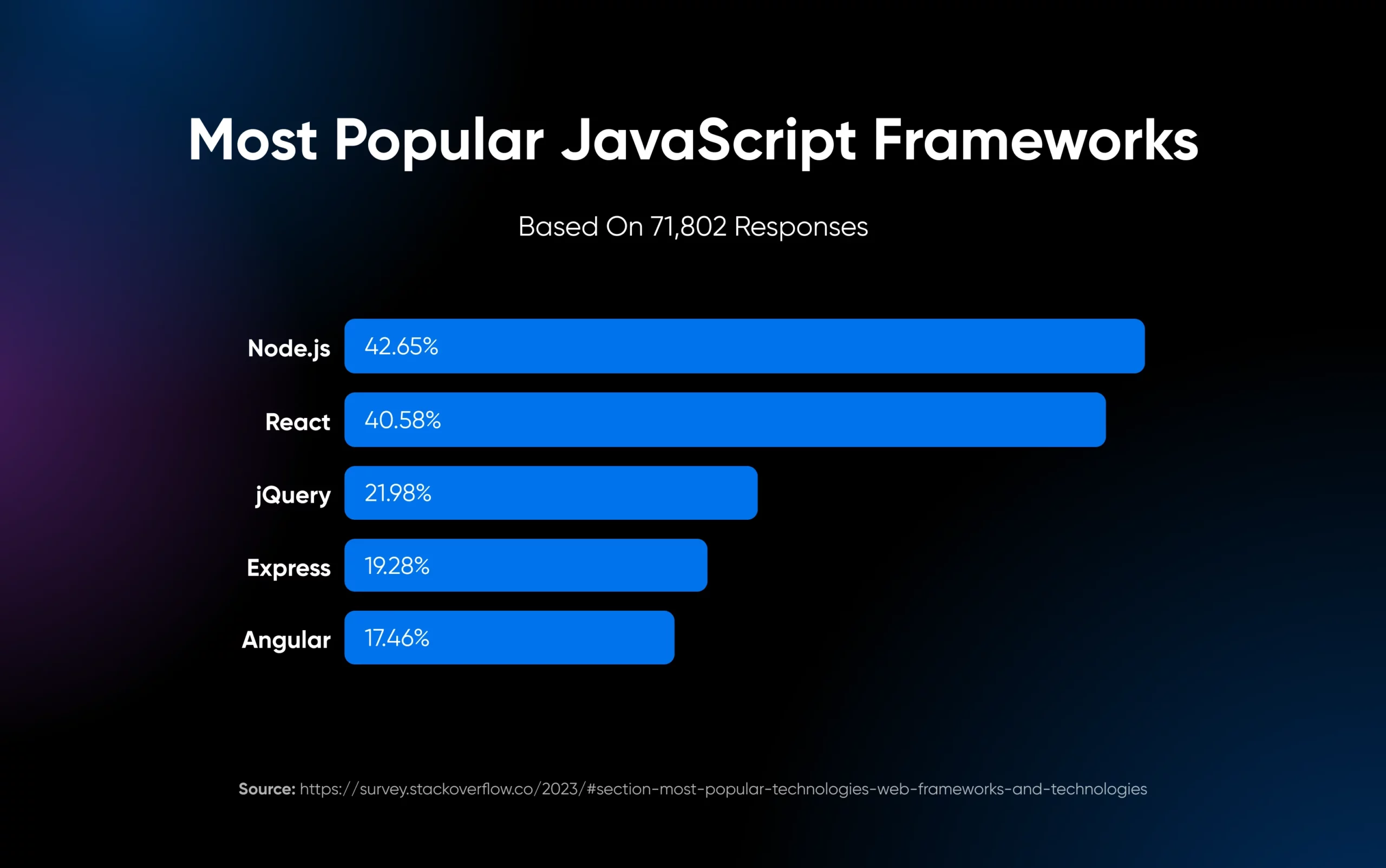
React can be used in building user interfaces, allowing developers to create interactive and dynamic web applications. React Native extends this to mobile app development. Facebook currently maintains React, along with a large community of developers who help keep it merrily humming along.
This library uses a virtual DOM and a component-based architecture instead of updating the entire webpage with every change.
Think of the virtual DOM as a simple copy of the actual web page’s structure.
When changes happen (like user input or data updates), React first updates this virtual DOM. Then, it figures out the best way to show those changes. It updates only the necessary parts of the actual web page. This approach makes rendering much faster, and the user experience is smoother.
Let’s take a look at a simple “Hello, world!” function of React.
Basic Structure Of A React Component
A key feature is the use of React components, including functional components. Consider these the building blocks of your UI. Each component is self-contained code representing a specific part of the interface.
Let’s look at a simple example.
import React from ‘react’;
function App(props) {
return (
<div className=”App”>
<h1>Hello React.</h1>
</div>
);
}
- For the above code, we’re first importing the React library.
- The “App” function takes a props (short for properties) as a parameter that can be passed to be used within the function.
- The content within the return() block is JSX.
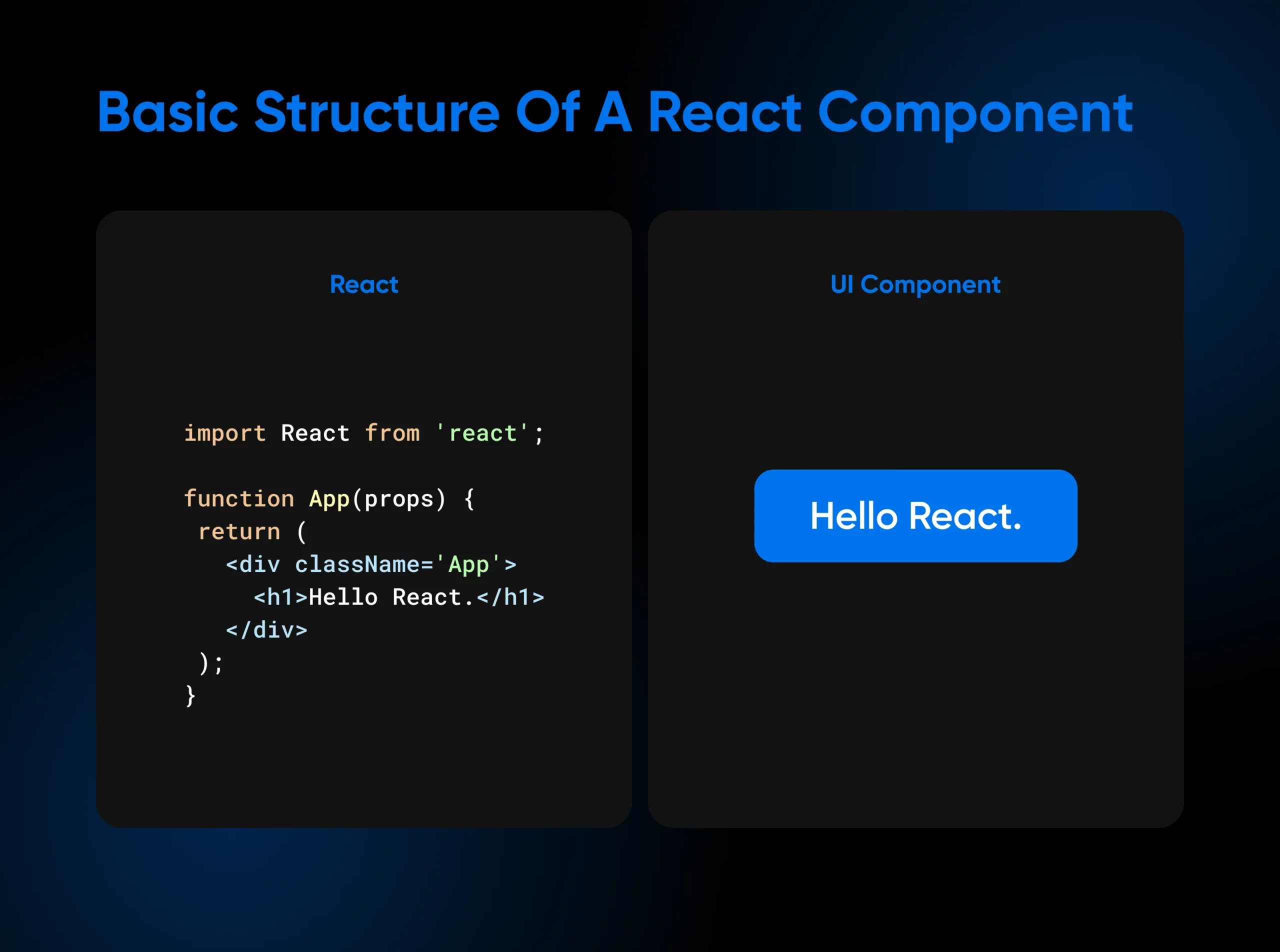
React uses JSX, a syntax extension that lets you write HTML-like code directly within your JavaScript files. This may seem unusual initially, but it provides a more visually intuitive way to define your UI elements and structure within your JavaScript code.
These features, along with a large and active community, make React a leading choice for developers building everything from single-page applications to complex web platforms.
Why You May Want To Learn React
React is currently enjoying a surge in popularity, and the trend points toward continued growth.
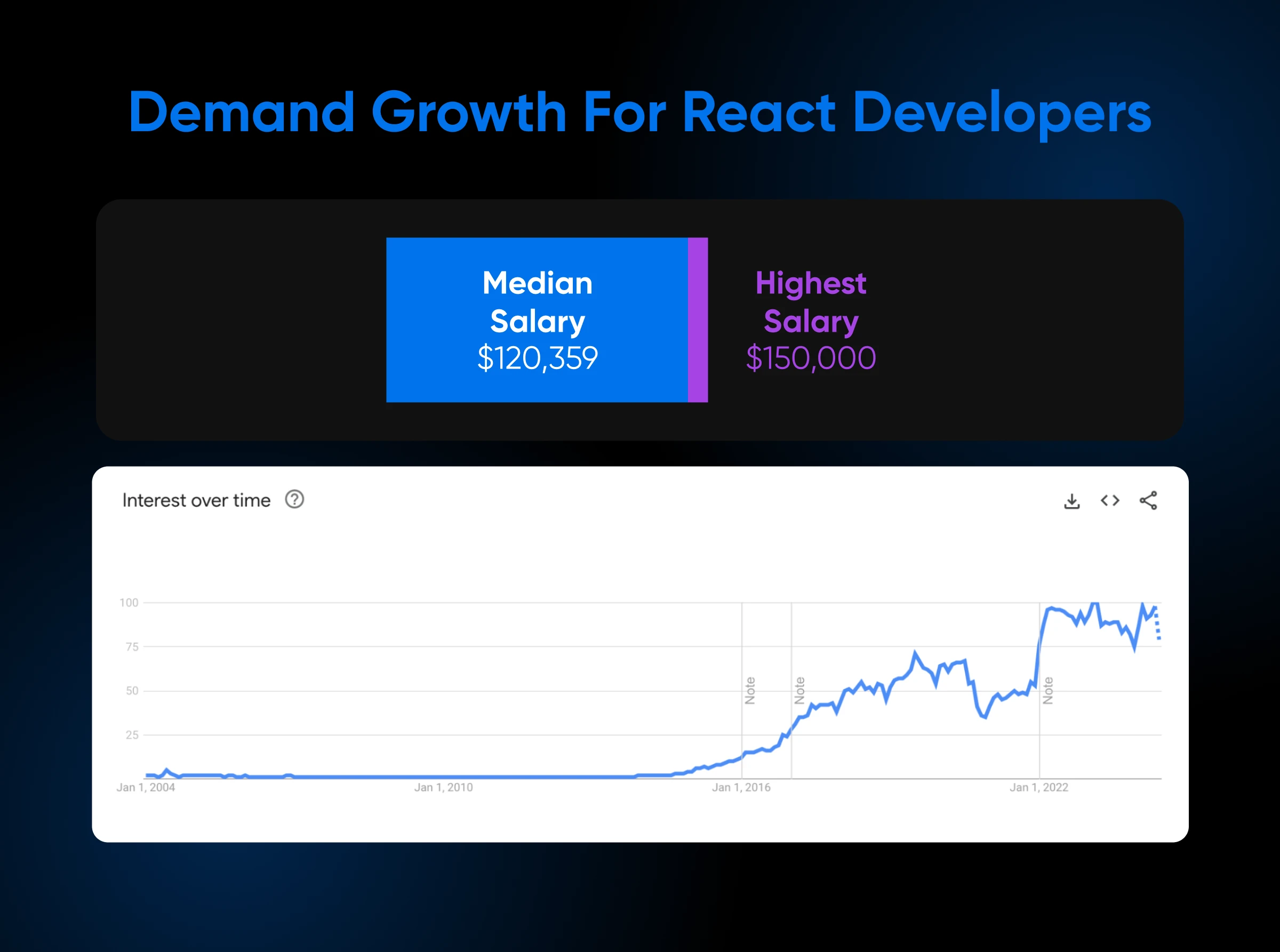
Front-end developers specializing in React can command average salaries of $120,359, often exceeding $150,000 annually for experienced developers, according to talent.com.
This popularity stems from the numerous benefits React offers to developers:
- Clean and maintainable code: React promotes clean, reusable code through its component-based structure and encourages the use of design patterns for efficient development. This approach is excellent, especially when tackling large projects, as it simplifies development and maintenance.
- Efficient debugging: Debugging becomes less of a headache with React. The framework helps developers focus on individual components, making it easier to pinpoint and fix errors.
- Enhanced performance: React’s virtual DOM improves performance, resulting in faster rendering times and a smoother user experience.
- Strong community and resources: React has a vibrant community of developers willing to lend a helping hand. It’s a goldmine of resources: tutorials, libraries, and support when you’re learning and beyond.
What sets React apart is its declarative approach. You don’t need to tell React how to update the UI step-by-step. Instead, you describe the desired outcome, and React handles the complex implementation details behind the scenes.
This efficient, streamlined approach to UI development is at the heart of React’s appeal, resulting in enhanced user experiences.
What To Learn Before React
Before you start learning React, you need a solid foundation in several web technologies. React itself is a JavaScript library. So, you will benefit from understanding JavaScript fundamentals to use it effectively.
This includes things like functions, objects, arrays, DOM manipulation, and ES6 syntax. Arrow functions, in particular, are commonly used in React code.
While React itself is a JavaScript library, diving into it requires a foundation in several core web technologies and concepts:
- Basic JavaScript: Learn the basics of JavaScript. Think functions, objects, arrays, and how to manipulate the DOM. Having a comfort level with ES6 syntax can help you speed up learning React.
- HTML and CSS proficiency: React relies on HTML and CSS for rendering and styling, so a strong understanding is a must. Want to make your applications look even better? Try exploring frameworks like Tailwind and Bootstrap.
- Version control with Git: Every developer, React-focused or otherwise, benefits from knowing Git. It’s about tracking changes, smooth collaboration, and the ability to rewind time on your codebase if needed.
- Basic understanding of package managers: Tools like npm or yarn are essential for managing the various libraries and dependencies within your React projects. Even a basic understanding of installation and management goes a long way.
A few other things can give you a head start, though they’re not strictly required. Webpack (or other module bundlers) can help organize your JavaScript code— Understanding its basics is beneficial as your project grows.
Similarly, Babel converts modern JavaScript code using advanced concepts into a format older browsers can understand. While not mandatory, people frequently use Babel with React to ensure cross-browser compatibility. This may seem like a lot, but don’t worry —There are many resources to help you learn these foundational technologies.
How To Learn React Fast (9 Methods)
If you’re interested in learning React, a handful of excellent resources will help streamline the process. We’ve compiled a list of the most useful and inexpensive options.
1. React Official Website
The official React docs are a complete resource for learning about this JavaScript library. You’ll find tutorials, examples, and helpful documentation. There’s also a community forum to connect with other React developers and ask questions.
Start with the “Learn React” section for a comprehensive step-by-step guide to mastering the library. This section progresses from basic to advanced concepts.
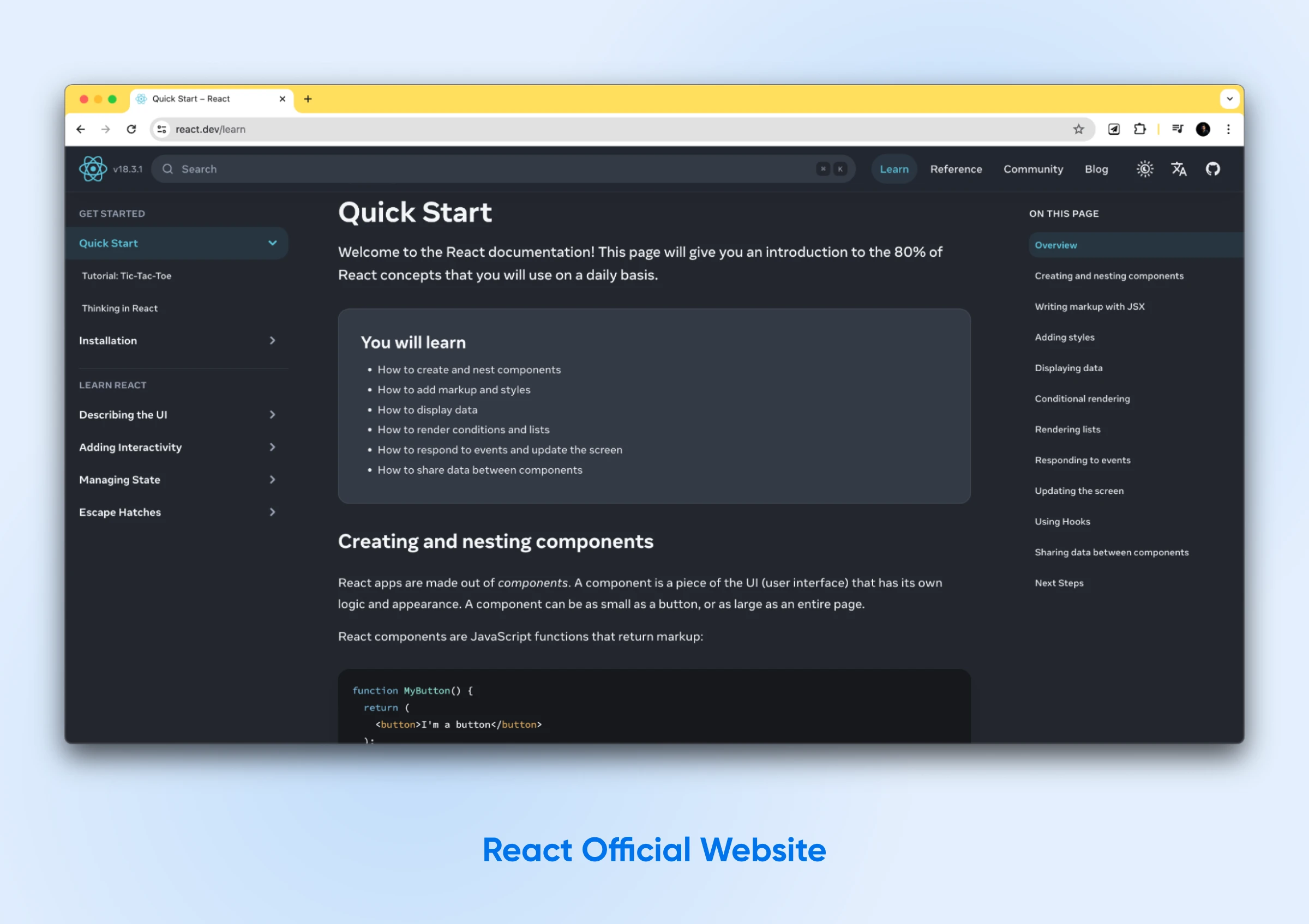
If you learn best by doing, this is a great place to start. You’ll learn fundamental concepts like components, props, and state. The documentation also covers the most essential React development techniques and thoroughly explains React’s benefits.
The website’s “Docs” tab houses a wealth of resources, tools, and articles categorized by specific topics and goals. You can find information about adding React to an existing website, using it to create a new application, or exploring advanced concepts.
2. Codecademy
Codecademy is a website that offers interactive courses on various programming languages, like React:
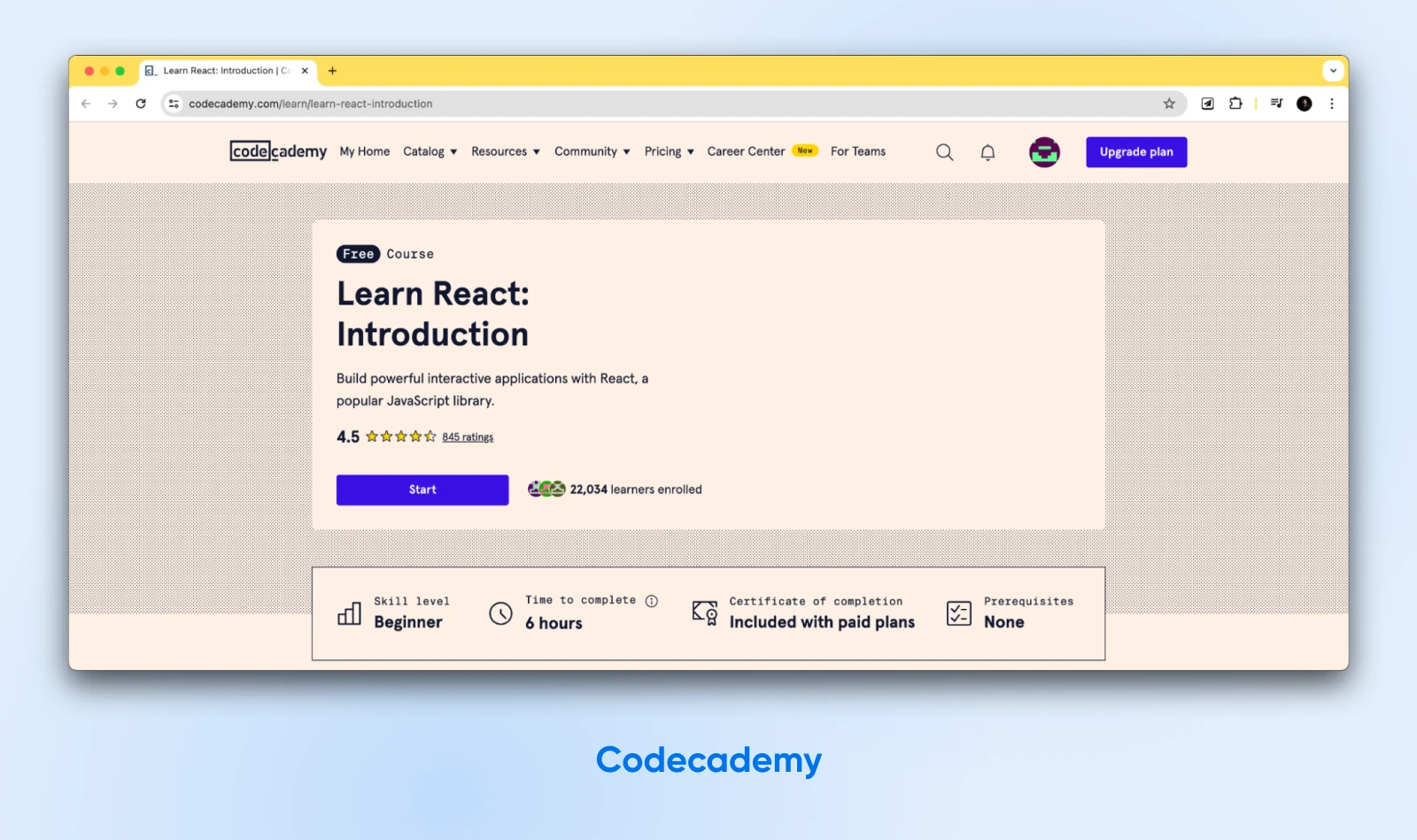
The courses are self-paced, so you can work through them at your own speed. More specifically, Codecademy offers a Learn React course on building front-end applications, including advanced concepts like implementing time travel functionality:
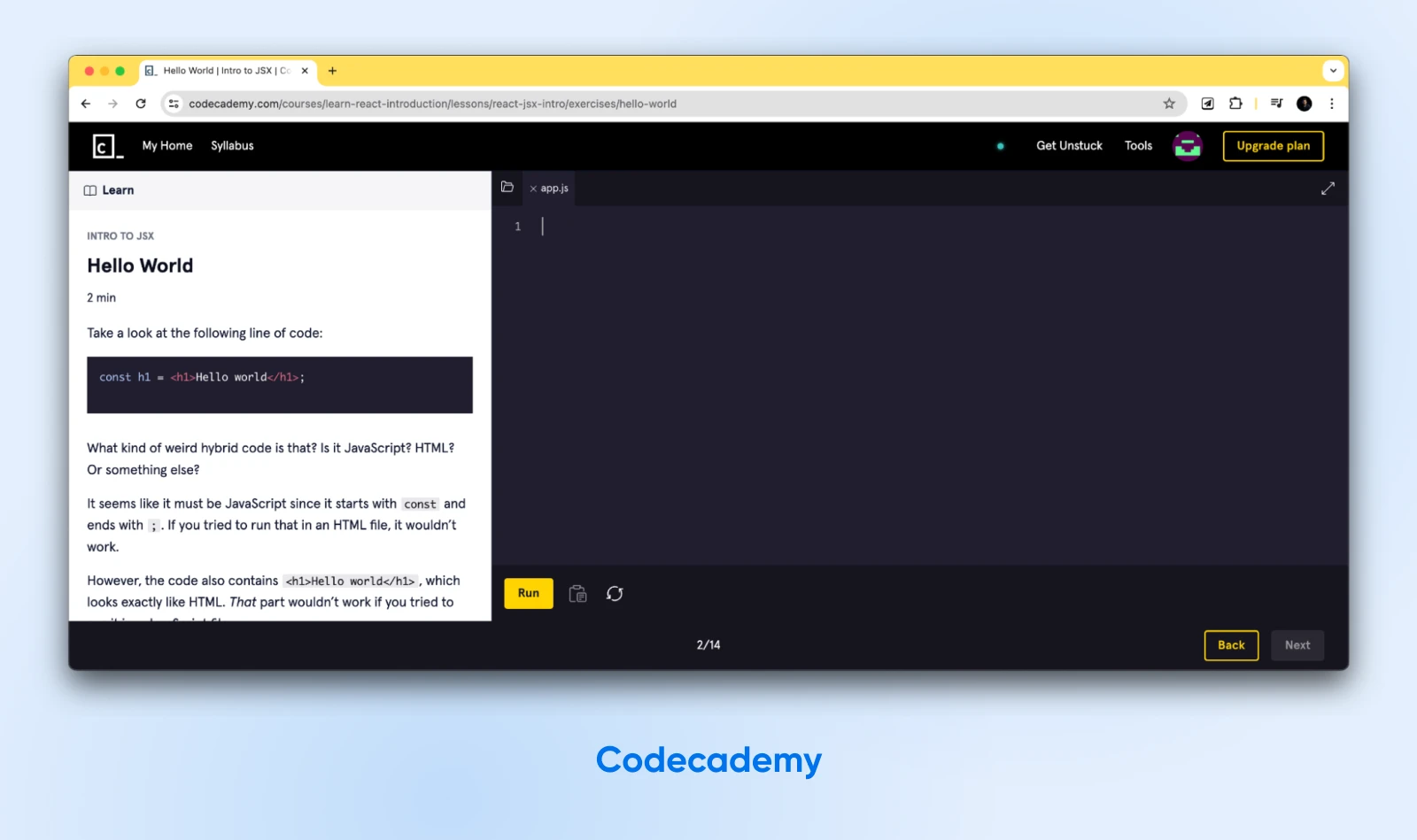
The Codecademy React course covers the basics of React, including how to create components, work with props and state, and leverage React hooks for enhanced functionality. Additionally, the course teaches you how to use React with JavaScript and how to build a simple front-end application with React. After you’ve completed this course, you should be able to build React applications confidently.
The course is free to take, but there is a monthly subscription fee if you want access to the full range of features. With the pro plan, you can earn a certificate of completion. It takes approximately 20 hours to complete.
3. FreeCodeCamp.org
FreeCodeCamp.org offers a cost-effective way for aspiring developers to learn React.
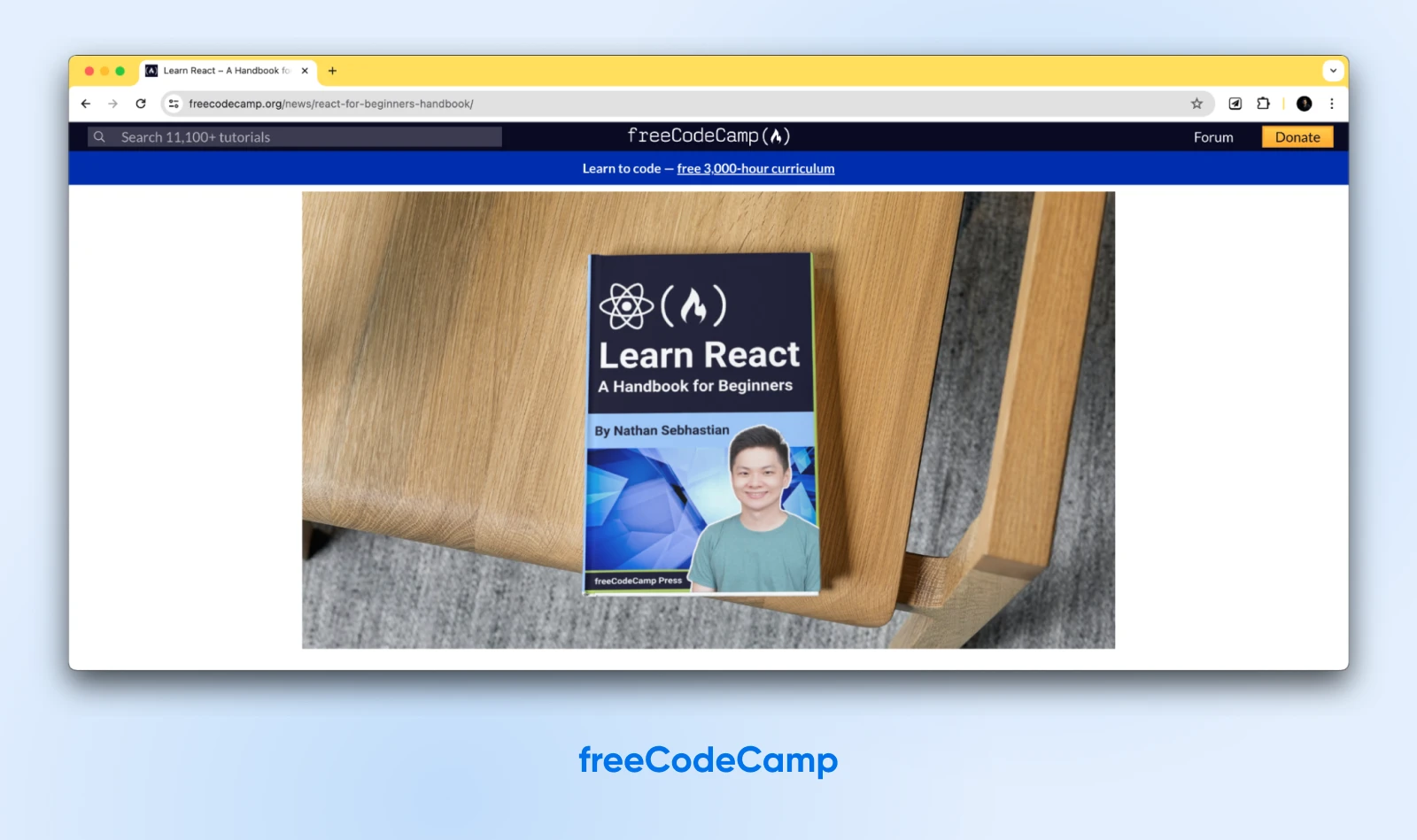
The platform excels at guiding beginners through foundational concepts before advancing to intermediate and advanced topics. Think of it as a personalized roadmap for your React learning journey. FreeCodeCamp provides a wealth of tutorials and resources to support every stage of development.
4. Udemy
Udemy is a popular platform that provides a wide range of online learning paths on various subjects. It offers over 3,000 courses on React alone. While some are outdated or short, there are plenty of solid options worth checking out, such as React JS Frontend Web Development for Beginners.
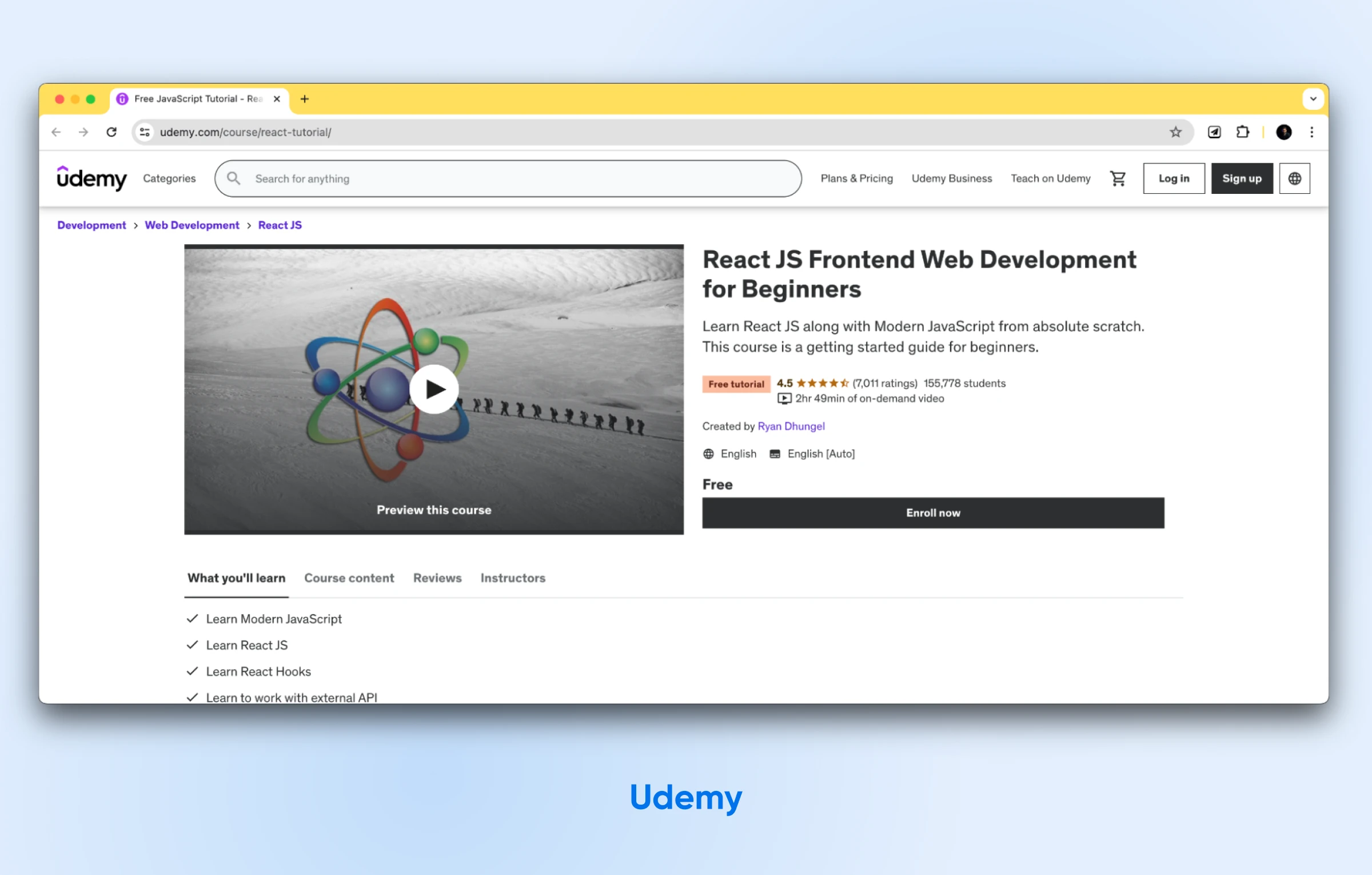
This free course teaches you the basics of hooks and working with external Application Programming Interfaces (APIs). It can also show you how to make AJAX requests and how to build a news app.
Udemy’s free courses all include nearly three hours of online video content. However, paid memberships are also available. With a paid plan, you can get a certificate of completion, plus instructor Q&As and direct messages.
5. Egghead.io
Egghead.io is another excellent resource for learning React. It offers video courses on React topics, ranging from beginner-friendly tutorials to advanced concepts. One of the most popular courses to learn React is The Beginner’s Guide to React:
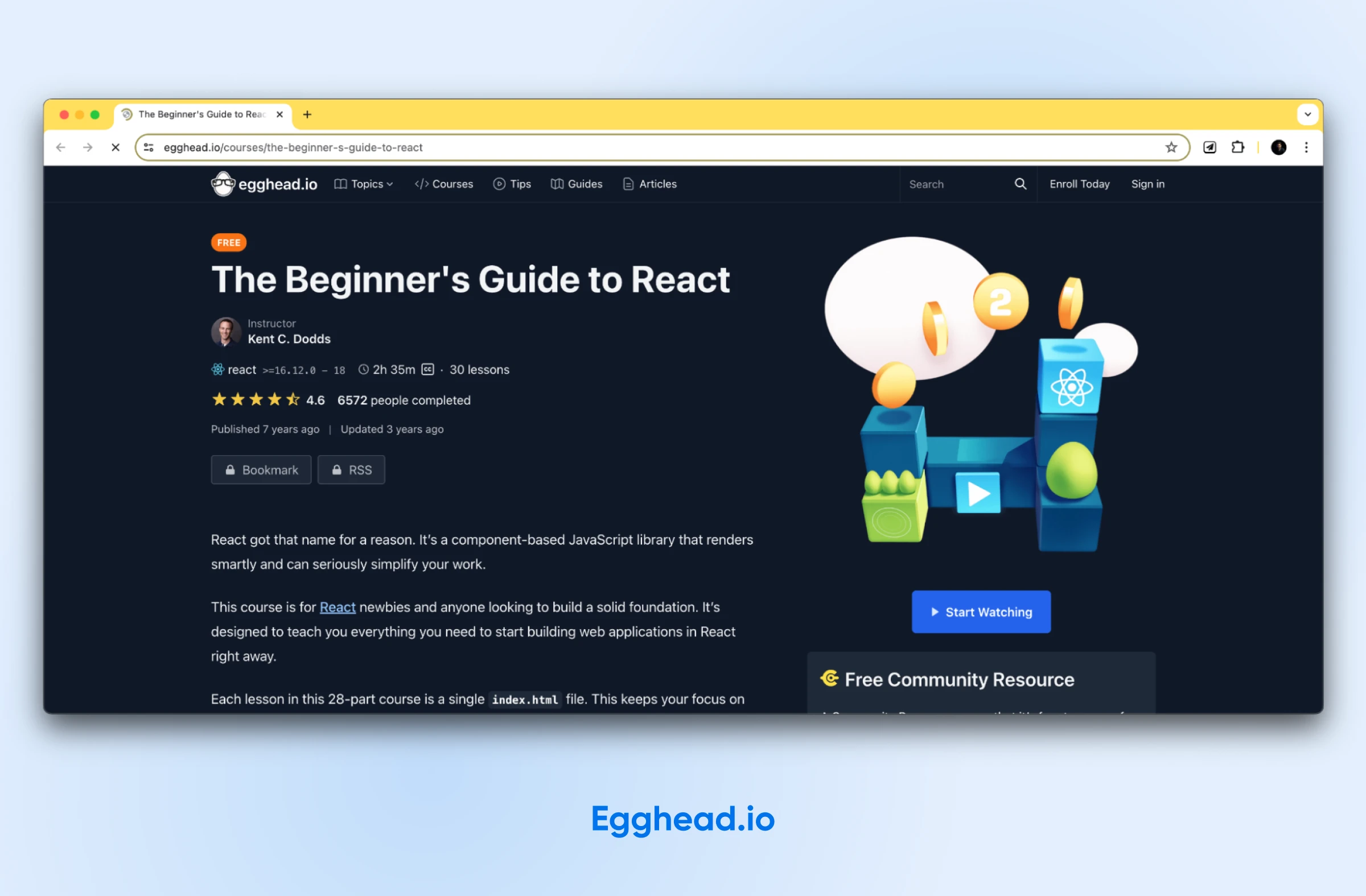
This free, hands-on course teaches you how to build smart websites with ReactJS. The course is composed of 28 parts, with each lesson in a single index.html file. It provides a distraction-free learning environment that lets you develop your skills in a focused, streamlined manner.
The course begins with a blank file and then gradually becomes more complex as you progress through the lessons. In the end, you’ll learn how to move to a product-ready environment and deploy your React apps. Additionally, the course teaches you what problems React can solve and how to work them out.
It also explains what JSX is and its role in standard JavaScript objects and function calls. In this course, you’ll also learn how to manage state with hooks and build forms.
6. Coursera
Another popular online platform for learning React is Coursera. The professional courses on this website are created by highly accredited universities and organizations worldwide.
You can start with the React Basics course by the creator of React, Meta:
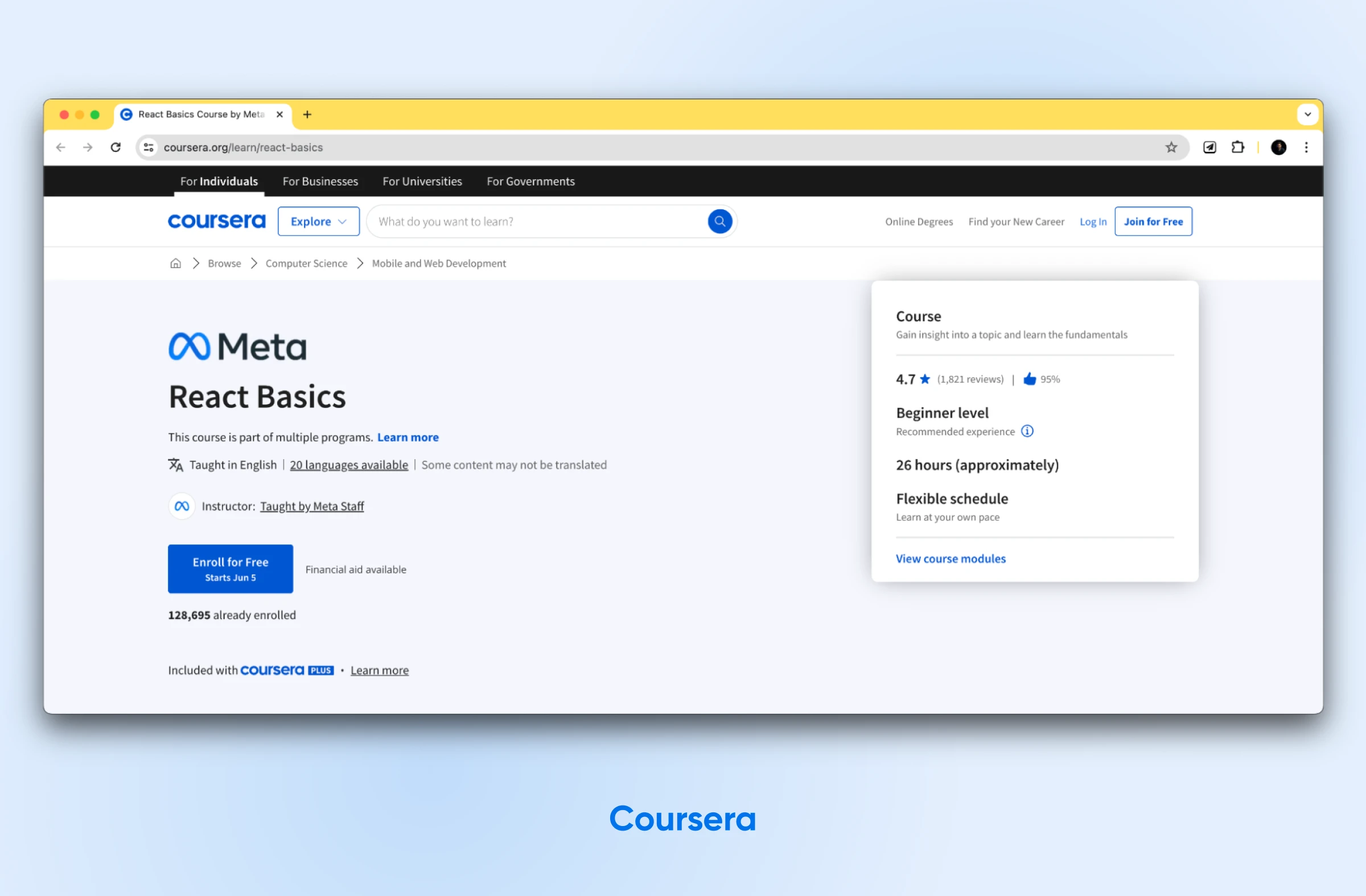
This course delivers an excellent introduction to React. Here are some key features of the course:
- Requires no prior coding experience, only basic internet navigation skills.
- Covers key concepts like component-based architecture, data flow with props, and building user interfaces with forms.
- Includes quizzes to test your understanding and 26 hours of flexible learning at your own pace.
- Gives you a shareable certificate upon course completion to showcase your new skills.
This course is particularly valuable because it’s taught by Meta staff and offers insights into real-world React development practices. While it doesn’t cover advanced concepts, it provides a strong foundation for further learning.
7. Scrimba
Scrimba is a powerful platform for learning React. It offers thousands of paths and courses to help you learn React Native, React app building, and much more.
One of the best Scrimba courses to learn React is aptly named Learn React:
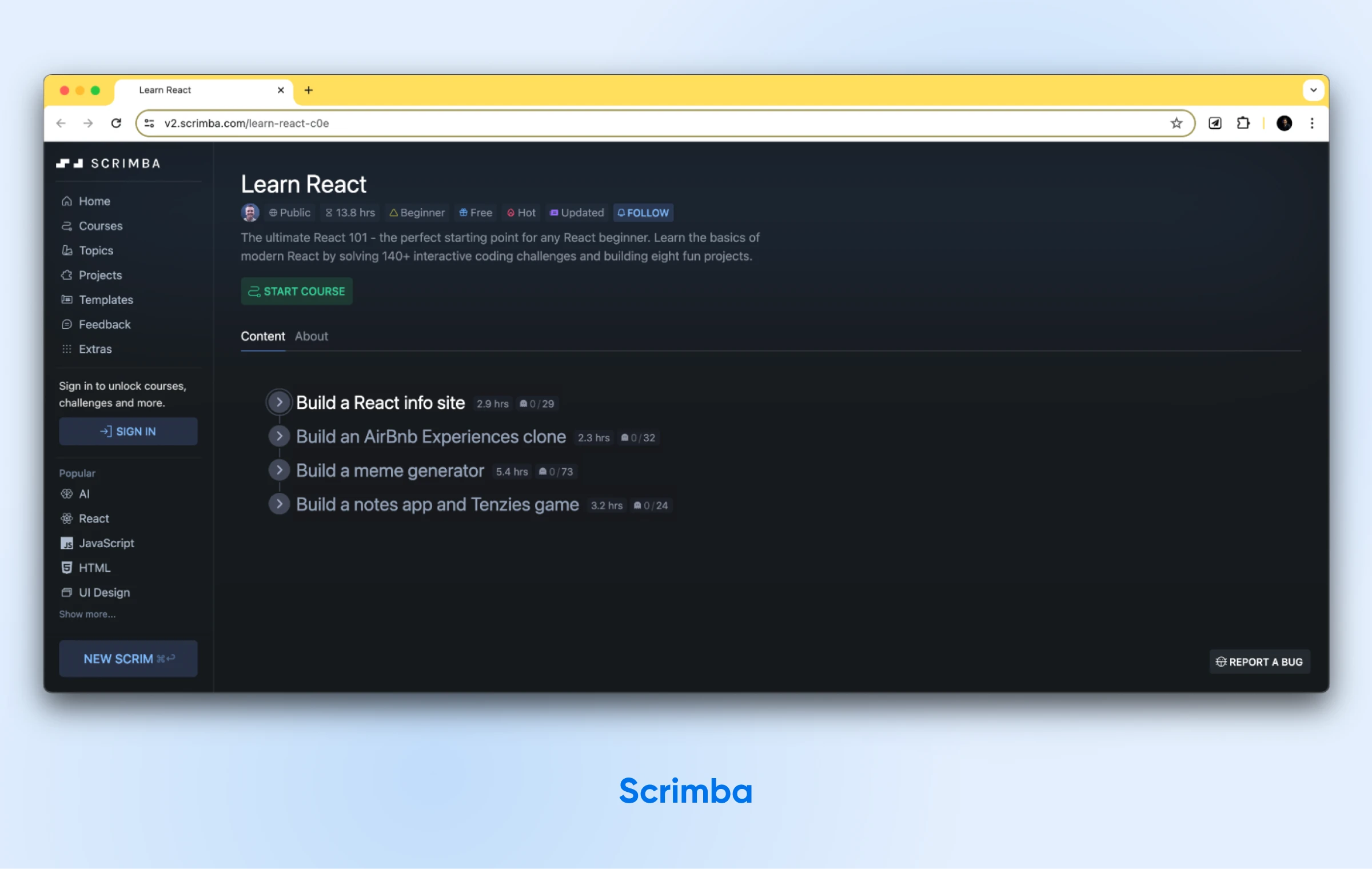
This interactive course is an excellent resource for beginners. It teaches the basics of modern React and offers lessons that require you to solve more than 140 coding challenges. You’ll build eight projects and explore 147 screencasts across four modules.
Throughout the course, you can take multiple paths. For example, you can learn how to build a React information site in two and a half hours. You can also learn how to build a meme generator or create an Airbnb experience website.
8. Facebook Create-React-App
Facebook’s create-react-app is a tool for creating a boilerplate React application:
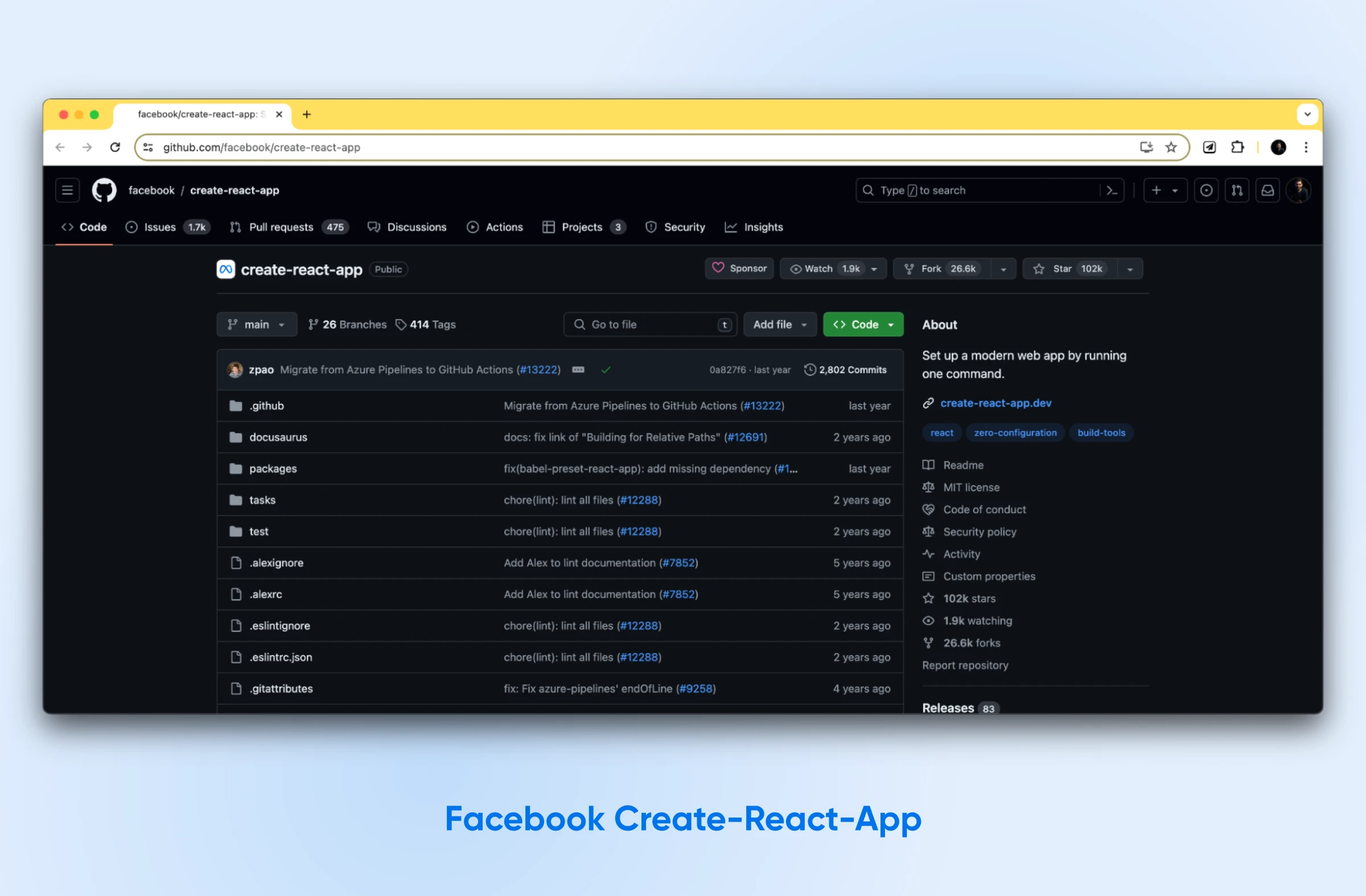
Available on GitHub, this resource for developers lets you get started with React quickly. It teaches you how to create a new app and develop apps bootstrapped with it, with no build configuration.
You can use it on macOS, Windows, and Linux. It’s completely free, and you don’t need to worry about installing or configuring tools, such as Webpack or Babel. You can simply create a project to get started.
9. YouTube Courses
YouTube is a fantastic, free resource for learning React. It offers an extensive collection of video tutorials and some full courses. Many seasoned developers and passionate educators come here to share their knowledge.
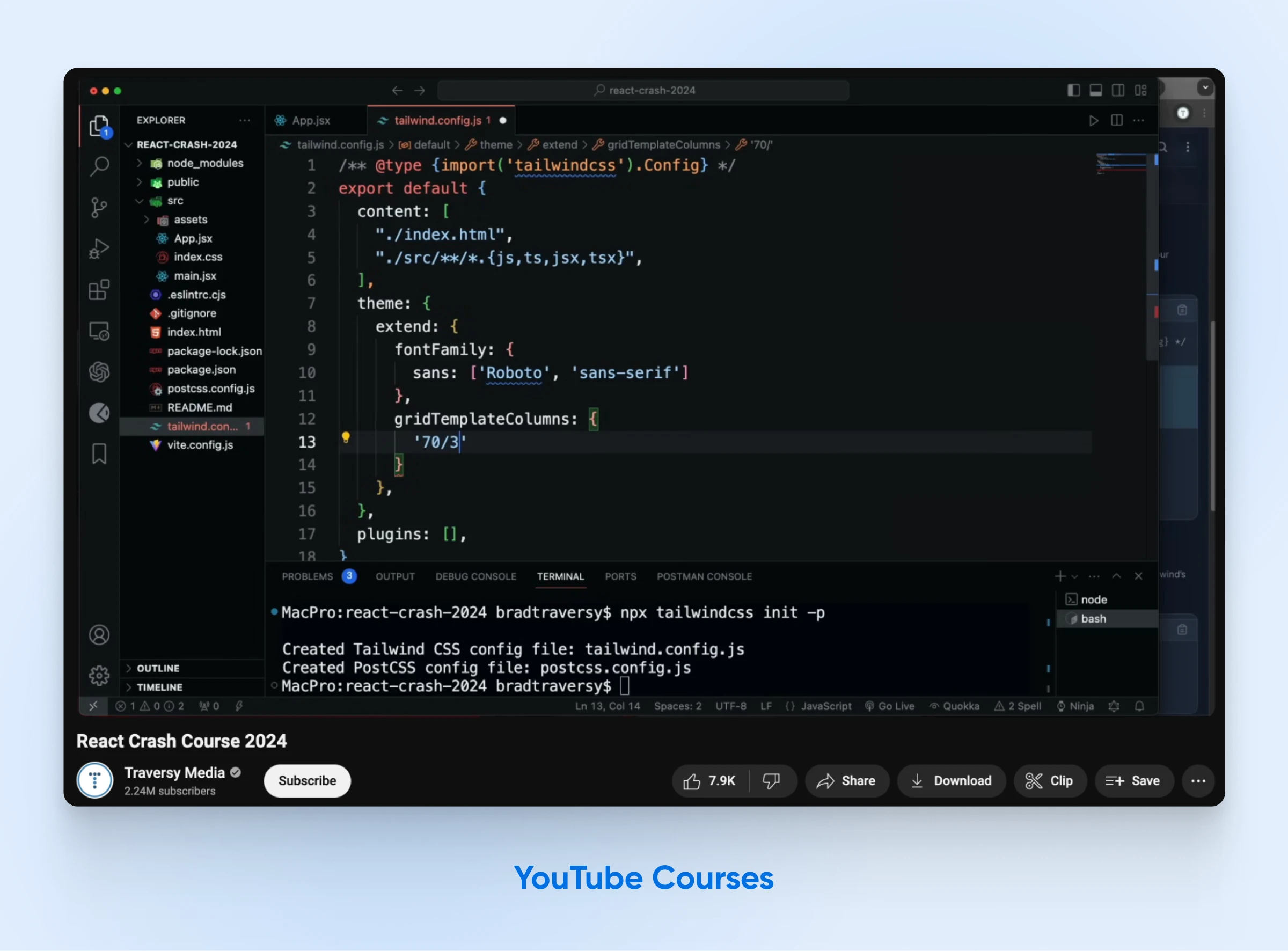
They present complex material in a digestible format to help beginners learn React concepts.
Let’s explore some top-tier YouTube channels and courses for learning React:
- Traversy Media’s React Crash Course: Brad Traversy, a well-known figure in web development instruction, presents this crash course on React. It quickly introduces beginners to the fundamentals of this library with practical examples and projects.
- The Net Ninja’s React Playlist for Beginners: The Net Ninja YouTube channel is known for its accessible teaching style. Their beginner-friendly React tutorial focuses on the essential concepts of React: components, state, and props. You’ll work on projects that solidify your understanding.
- Codevolution’s React Playlist: Codevolution provides a detailed walkthrough of React, examining its core concepts and features. Hands-on coding exercises and projects reinforce your learning throughout the course.
These YouTube courses and channels equip you with the knowledge and practical examples needed to learn React so you can start creating applications in no time.
What Are The Challenges Of Learning React?
Learning React comes with its share of hurdles, even for experienced developers.
To start with, switching to component-based architecture and a declarative UI demands a whole new way of thinking about application development. You’ll need to master concepts like JSX, props, state, and lifecycle methods — they’re the backbone of React.
Adding to this is the sheer size of the React ecosystem. While the variety is great for flexibility, the number of libraries, tools, and potential architectures can feel overwhelming. Choosing the right approach for your project becomes a challenge in itself.
Then, there’s the world beyond the core library. You’ll likely encounter tools like Redux for state management and Webpack for bundling, each with its own learning curve. Successfully weaving these elements into a cohesive application architecture requires a separate set of skills.
Despite these challenges, React’s component model leads to more manageable and reusable code. The initial learning curve, while steep, often proves worthwhile for developers seeking to build robust and maintainable user interfaces.
How To Pick The Right Learning Resources For React?
To learn React well, you’ll want resources that fit how you learn best. It’s also good to mix structured lessons with hands-on practice. Still trying to figure out where to start? Here are a few ideas:
- Platforms like Codecademy and Scrimba are great if you learn by doing. They offer coding exercises with instant feedback, so you can see if you’re on the right track.
- If videos are your thing, check out Egghead.io, Udemy, or even React’s own website. They have comprehensive courses that walk you through everything.
- Sometimes you want explanations you can read carefully. For that, look to the React documentation itself, or sites like FreeCodeCamp, CSS-Tricks, and Smashing Magazine. They’re full of helpful guides and in-depth articles.
- If you want something structured and free, YouTube may be your best bet. Your only task will be filtering out the outdated and not-so-good tutorials.
The best approach is generally a combination of multiple learning methods. For instance, you could start with a YouTube beginner’s course and then take a full certification course. Once you’re ready, you’ll solve more complex problems by looking up community posts and documentation and maybe even asking community members.
Start Learning React Today
React gives you the power to build front-end applications. You can create complex user interfaces more efficiently and with less hassle than you might have experienced before. As you start building more complex React applications, you will need to find a dependable hosting platform to share your creations reliably with others.
Consider DreamHost for your hosting needs. DreamHost offers affordable and reliable shared hosting plans that are perfect for your React projects. You can focus on what you do best — building amazing user experiences — while we provide the speed, security, and support your projects need.
Start your React journey with DreamHost and take your projects to the next level.